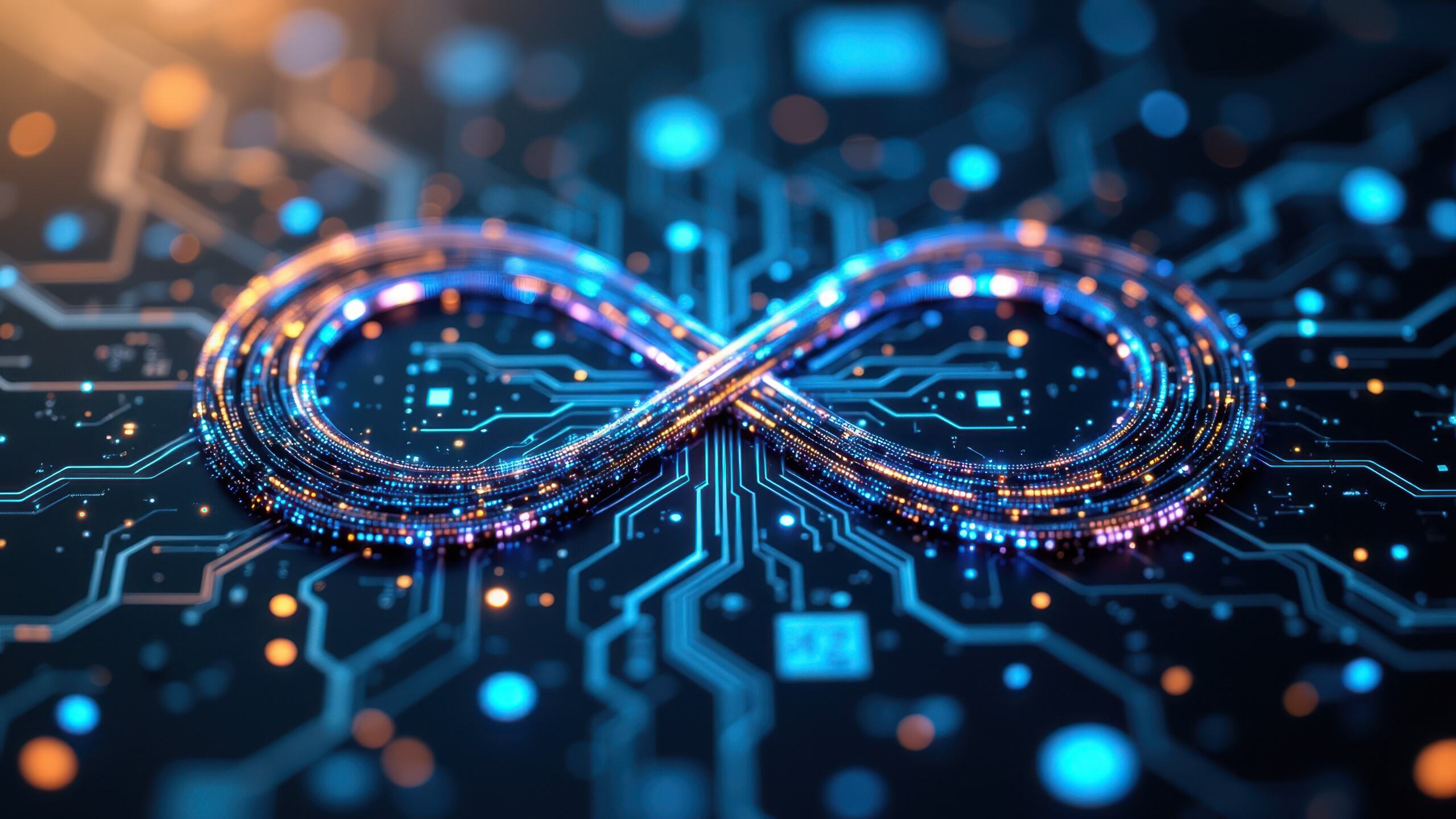
Managing nested categories in hierarchical data structures can be a challenging task, especially when you’re working with dynamic data like in e-commerce, content management systems, or organizational hierarchies. Each category can have subcategories, and these subcategories can further contain their own subcategories, leading to a complex tree-like structure. This blog post dives into how to efficiently manage nested categories using MongoDB and design APIs for handling such hierarchies.
The Problem: Managing Nested Categories
Consider an e-commerce platform where categories are organized into multiple levels, as demonstrated in the example code on GitHub.
Retrieving a category along with its subcategories requires managing a parent-child relationship that can become complex as you go deeper. Common challenges include:
- Depth of Hierarchy: Categories can nest to multiple levels, making it difficult to retrieve all subcategories efficiently.
- Data Consistency: Ensuring that categories maintain correct parent-child relationships is crucial, avoiding self-referencing or circular dependencies.
- Efficient Querying: Pulling all categories, including their nested subcategories, can result in significant performance overhead if not managed well.
Unlike SQL databases, MongoDB does not support recursive joins natively. Thus, nested categories require a different approach, typically using MongoDB’s aggregation pipeline.
The Solution: MongoDB Aggregation Framework
The nested category system can be modeled using MongoDB by having each category reference its parent category. Here’s a simplified version of the category schema:
const categorySchema = new Schema({
name: {
type: String,
required: true,
index: true
},
parent: {
type: Schema.Types.ObjectId,
ref: 'Category'
}
});
categorySchema.index({ name: 1, parent: 1 }, { unique: true });
In this schema, each category can have a parent, allowing the creation of an unlimited hierarchy. The challenge is to retrieve categories along with their nested subcategories.
Listing Nested Categories
To retrieve categories and their subcategories recursively, we use MongoDB’s aggregation pipeline with the $lookup stage to create a self-join on the same collection. Here’s how you can fetch categories and their children up to a specified depth:
app.get('/categories', async (req, res) =>
try {
let depth = parseInt(req.query.depthLevel) || 1; // Depth of the hierarchy
depth = depth <= 0 ? 1 : depth;
let limit = parseInt(req.query.limit) || 10; // Limit on the number of categories returned
// Generate the recursive lookup pipeline
let pipeline = await _generateNestedPipelines(depth, []);
pipeline.push({ $limit: limit });
const categories = await CategoryModel.aggregate(pipeline);
return res.status(200).json({
status: 'SUCCESS',
count: categories.length,
items: categories
});
} catch (error) {
return res.status(200).json({ status: 'FAILED', count: 0, items: [] });
}
});
The Recursive Pipeline
To handle nested categories, we define a helper function that builds the aggregation pipeline recursively, based on the desired depth of the hierarchy:
async function _generateNestedPipelines(depth, pipeline = []) {
// Add a lookup stage for the parent-child relationship
function addLookupStage(pipeline, depth) {
if (depth <= 0) return; // Stop recursion when depth is 0
pipeline.push({
$lookup: {
from: 'categories', // Reference the same collection
localField: '_id', // The current category's ID
foreignField: 'parent', // The parent field in the child categories
as: 'children', // Name of the array for subcategories
pipeline: addLookupPipeline(depth - 1) // Recursion for nested children
}
});
}
// Sort the categories and recursively add lookup stages
function addLookupPipeline(depth) {
const pipeline = [{
$sort: {
'name': 1 // Sort categories alphabetically
}
}];
addLookupStage(pipeline, depth);
return pipeline;
}
// Start by adding the initial lookup stage
addLookupStage(pipeline, depth);
return pipeline;
}
This pipeline performs a self-join on the categories collection, allowing you to retrieve the children for each category. The depthLevel query parameter controls how deep the recursion goes, ensuring that you can limit the performance overhead when querying deeply nested structures.
Example Query
To list categories along with their subcategories up to 3 levels deep, you would make a request like this:
GET /categories?depthLevel=3&limit=5
This request returns the top 5 categories, each with their nested children up to 3 levels deep.
Where You Might Encounter This Problem
The problem of managing nested categories isn’t limited to just e-commerce. Here are some areas where this pattern frequently appears:
- E-commerce Platforms: Categories like “Women” > “Clothing” > “Dresses” help users browse products efficiently.
- Content Management Systems (CMS): Blogs, articles, or media files are often organized into nested categories for easier navigation.
- Organizational Hierarchies: Corporate structures where departments and teams have sub-teams or regional divisions can be modeled using nested categories.
- Supply Chain Management: Products may be categorized into hierarchical groups such as “Materials” → “Metals” → “Aluminum.”
- Location-Based Data: Geographical hierarchies, such as “Country” → “State” → “City,” require recursive querying to display nested locations.
Challenges and Considerations
- Performance: Recursive lookups can be resource-intensive, especially for large datasets. Limiting the depth of recursion and the number of results returned can help mitigate performance issues.
- Data Integrity: The system ensures that categories cannot reference themselves or create circular parent-child relationships, which could break the nested structure.
- Handling Large Data Sets: For applications dealing with large numbers of categories, optimizing the aggregation pipeline and managing database indexes is crucial to maintain performance.
Conclusion
Handling nested categories in MongoDB requires careful design, especially when dealing with recursive relationships. By leveraging MongoDB’s aggregation framework and $lookup stage, you can efficiently retrieve deeply nested categories while maintaining control over depth and performance. This approach is flexible, allowing you to scale as your data grows in complexity, making it well-suited for e-commerce platforms, content management systems, and organizational hierarchies.
However, to further optimize performance and reduce database load, consider implementing server-level caching. Here are some caching strategies:
- In-Memory Caching (e.g., Redis): Store frequently accessed category data in an in-memory store like Redis. This significantly reduces query load on MongoDB, allowing for faster response times.
- Application-Level Caching: Use caching mechanisms within your application framework (like Node.js with node-cache or express-cache) to store nested category results temporarily. This can be especially effective for pages where categories don’t change frequently.
- Cache Expiry Strategies: Implement expiration policies to refresh cached data periodically, ensuring users see up-to-date information. For example, cache category structures for an hour and refresh if there are updates.
Adding server-level caching can greatly improve response times and reduce MongoDB query overhead, providing a smoother user experience without compromising data accuracy.